Lesson#194: Make an area calculator for Triangles, Rectangles, and Circle in VBA
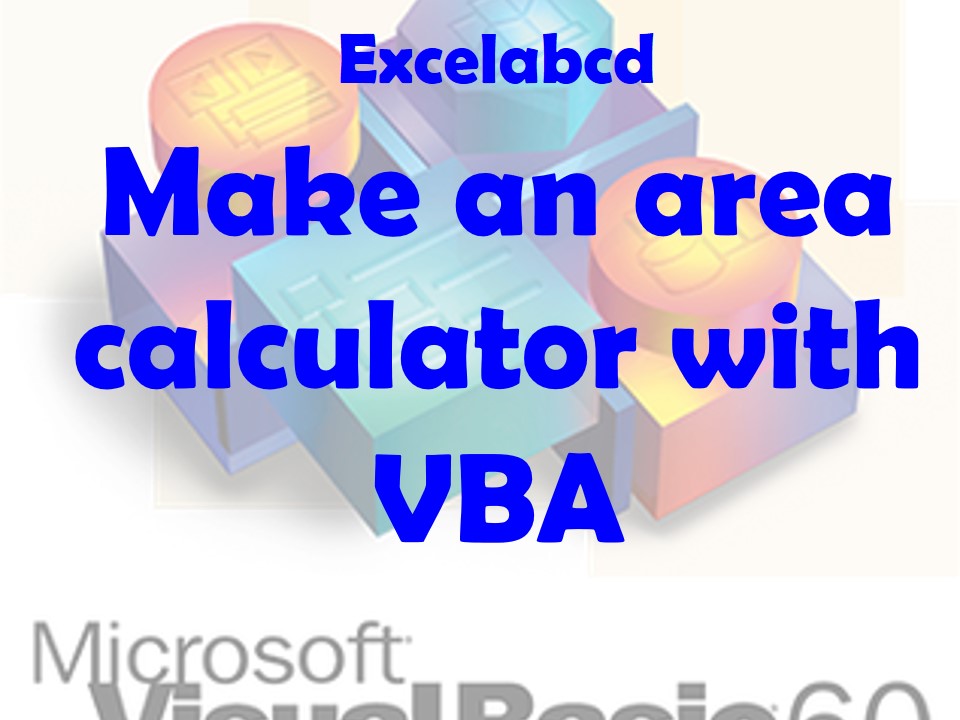
- Go to the Developer Tab in Excel. If you haven’t activated the Developer Tab then see here how to do it.
- Insert a Command Button by clicking on ‘Insert’.
- Create a button on the sheet and double-click to enter the code.
To create an area calculator in Visual Basic for Applications (VBA) that can calculate the area of a triangle, rectangle, or circle, you can use the following code:
Private Sub CommandButton1_Click()
Dim shape As String
Dim area As Double
shape = InputBox("Enter the shape (triangle, rectangle, or circle):")
Select Case shape
Case "triangle"
Dim base As Double
Dim height As Double
base = InputBox("Enter the base of the triangle:")
height = InputBox("Enter the height of the triangle:")
area = (base * height) / 2
Case "rectangle"
Dim length As Double
Dim width As Double
length = InputBox("Enter the length of the rectangle:")
width = InputBox("Enter the width of the rectangle:")
area = length * width
Case "circle"
Dim radius As Double
radius = InputBox("Enter the radius of the circle:")
area = 3.14159 * radius ^ 2
Case Else
MsgBox "Invalid shape."
End Select
If area > 0 Then
MsgBox "The area of the shape is: " & area
End If
End Sub
This code defines a command button that, when clicked, prompts the user to enter the shape for which they want to calculate the area. The shape is then passed to a Select Case
the statement, which determines the appropriate formula for calculating the area based on the shape. The area is then calculated and displayed in a message box.
To use this code in an Excel spreadsheet, you will need to create a command button and attach the event handler to its “Click” event. You can then test the calculator by entering different shapes and observing the resulting area calculations.
I hope this helps to give you an idea of how to create an area calculator in Excel using VBA. Let me know if you have any other questions or if you need further assistance.
Leave a Reply